We have already seen what Props in React are, in our previous blogs when we compared props and states and how to use them in functional and class components.
In this blog, we will understand how important props are in React when it comes to making the flow of our app dynamic.
Let’s begin…
What are props in React?
Props are the short form of properties that we can pass between the parent and the child component and can use it as we want but cannot change its values as they are read-only properties.
As told above, they can easily make the flow of the app dynamic
We can pass everything as props to the child component, especially functions that will help in updating the state of the parent component.
Let’s understand props with a simple React code –
We will make two reusable components Example.js and Tool.js, where the Example Component is the parent Component, and the Tool Component is the child component as shown
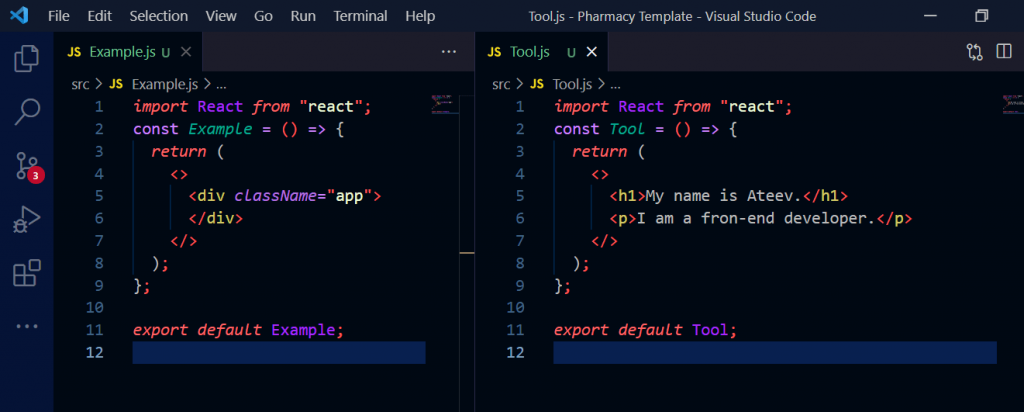
Now, import the Tool Component in the Example Component and pass a prop – name=”Ateev” in the with the Tool Component.
import React from "react";
import Tool from "./Tool";
const Example = () => {
return (
<>
<div className="app">
<Tool name="Ateev" />
</div>
</>
);
};
export default Example;
And we will use this prop in our Tool Component like this
import React from "react";
const Tool = (props) => {
return (
<div className="bg-dark text-white">
<h1 className="px-5 pt-5">My name is {props.name}.</h1>
<p className="fs-5 px-5 pb-5">I am a front-end developer.</p>
</div>
);
};
export default Tool;
We will get something like this –

We have passed the name from the Example Component as props to the Tool Component where we have used this in our Tool Component as props.name as shown, and we have our value in the above.
There are various ways of passing the props and using them inside our child component.
Let’s first discuss how we can use props in our child component. There are mainly three ways of doing it –
- With destructuring
- Without destructuring
- Using defaultProps
Using Props in React Without Destructuring
The above example is the perfect case of using props without destructuring them first.
We have to use props.name every time we have to use props in our child component and do not forget to use them inside the curly braces ({}).
We also have to declare its fist at the very beginning of our function in the brackets () as shown
import React from "react";
const Tool = (props) => {
return (
<>
</>
);
};
export default Tool;
This method is not general when it comes to using props. Every time we have to add the props word as a prefix which sometimes can get confusing if we are passing events or functions.
Using Props in React With Destructuring
It is the most common method to use props in the child component. We don’t have to add the word props as the prefix for every prop we use.
For the above example, let’s destructure our prop in the child component for ease of use
import React from "react";
const Tool = ({ name }) => {
return (
<div className="bg-dark text-white">
<h1 className="px-5 pt-5">My name is {name}.</h1>
<p className="fs-5 px-5 pb-5">I am a front-end developer.</p>
</div>
);
};
export default Tool;
The method to pass the props will remain the same, the only change is in our child component or Tool Component.
As you can see, we have destructured the name prop as ({name}). With this done, we can use the propname in our child component as it is without the prefix – props as shown in the above code, and there will be no change in the output of our component.

This method is only beneficial when there are a small number of props like say 5 or 6 at most, if in any case, the value of the props passed from hr parent to the child component is more than 6, we cannot destructure all the values as it will be time-consuming and we use React to minimize our time, we have to the props without destructuring it as it will be the optimal way to save time and effort.
Using Default Props in React
What if we have a set of data in which all the propname are not the same as shown –
import React from "react";
import Tool from "./Tool";
const Example = () => {
return (
<>
<div className="bg-dark text-white px-5 py-5">
<Tool name="kapil" eyeColor="blue" age="23"></Tool>
<Tool name="Sachin" eyeColor="blue"></Tool>
<Tool name="Nikhil" age="23"></Tool>
<Tool eyeColor="green" age="23"></Tool>
</div>
</>
);
};
export default Example;
We still have our Tool Component as our child component. In the above example. We are passing name, eye color, and age as props to our Tool Component, but some props are missing in all the above cases.
import React from "react";
const Tool = (props) => {
return (
<>
<div>
<p> Name: {props.name} </p>
<p>EyeColor: {props.eyeColor}</p>
<p>Age : {props.age} </p>
<hr></hr>
</div>
</>
);
};
export default Tool;
Below is the result of the Example Component
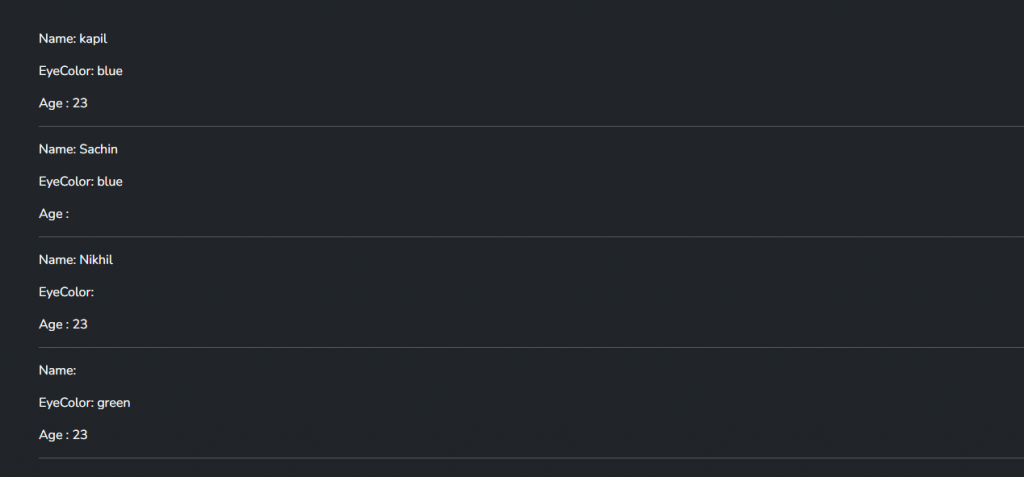
Notice that some values are missing in the output. They are only missing because we have not passed them as props in our Example Component.
If a situation like this comes where we dont have a value for some props, but we have it for the other, default props are used in this situation.
As the name suggests, these are the default values for the propname that we have passed in our Child Component.
It is passed in the child component after the export default function or at the end of our component and is passed as an object with key and value pairs.
Tool.defaultProps = {
name: "Atul",
eyeColor: "red",
age: "45",
};
After writing this in our Tool Component, the output will be –
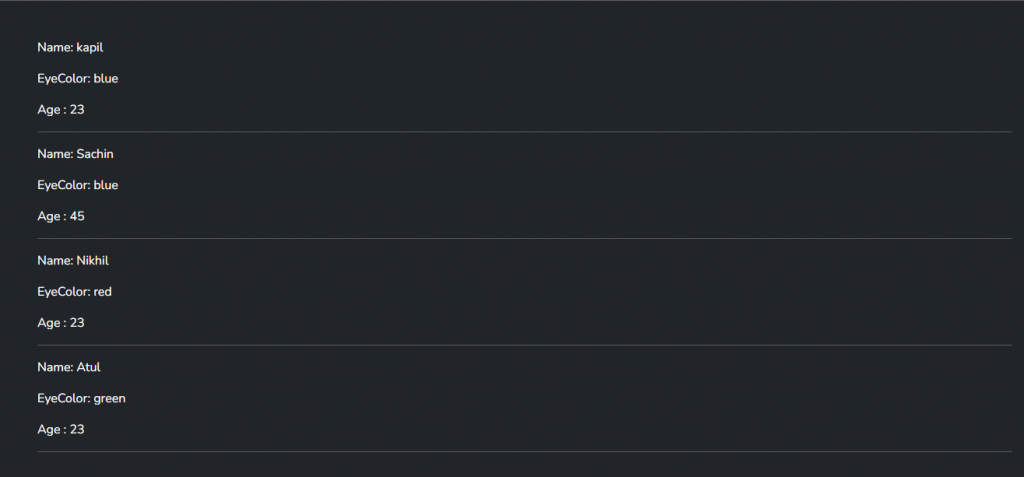
There are no empty spaces in our output this time as we have given a default value for all the props we have passed in the Tool Component.
In any case, any prop has not been passed from the parent to child component, but the child component is using that prop, then that prop will show its default value.
Click here to see different use cases in which we can pass props from the parent component to our child component.
Conclusion
Props are the read-only properties that are passed between two components mostly from the parent component to the child component.
There are three ways in which we can use props in our child component, and we have discussed them in this blog.
But, there is only one problem with this i.e we are not telling our App what type of prop it is, and then also we are getting our result correctly.
This can become a major problem when we make a large app. We simply cannot pass any type of data, this will result in an error, and in large apps, it’s not easy to find one out. For this specific purpose, React has given us proptypes through which we can do a type check of the values passed and if the values do not match, we will receive an error msg in the console