We generally write our code in React using one of the two components that are either functional or class components, but we don’t know the actual difference between them?
In this blog, we will uncover their differences and also which one is superior and why?
What is a component?
Components are just the parts of our UI that we have created for developing and debugging our Apps. It is easy to create one and attach it to the main component. We can create it very easily just by following the naming conversion.
Through this, error spotting and debugging becomes child play as we can deal with that separately without disturbing the rest of the code.
They are also reusable, as in you can easily use the same piece of code on any page or the same page without writing it again for that area. Simply put, it follows the well-known DRY principle.
State and Props
Components are like JavaScript functions that need an arbitrary input like state and props and return the React element for that specific task.
So, what is the difference between the two?
Difference between State and Props
A state is just a plain JavaScript object that React uses to store the initial value of the Component and will be used internally in the component.
Props are states that can pass its value from the parent Component to the Child Component
Let’s understand the difference between them and how they are called within the component(s).
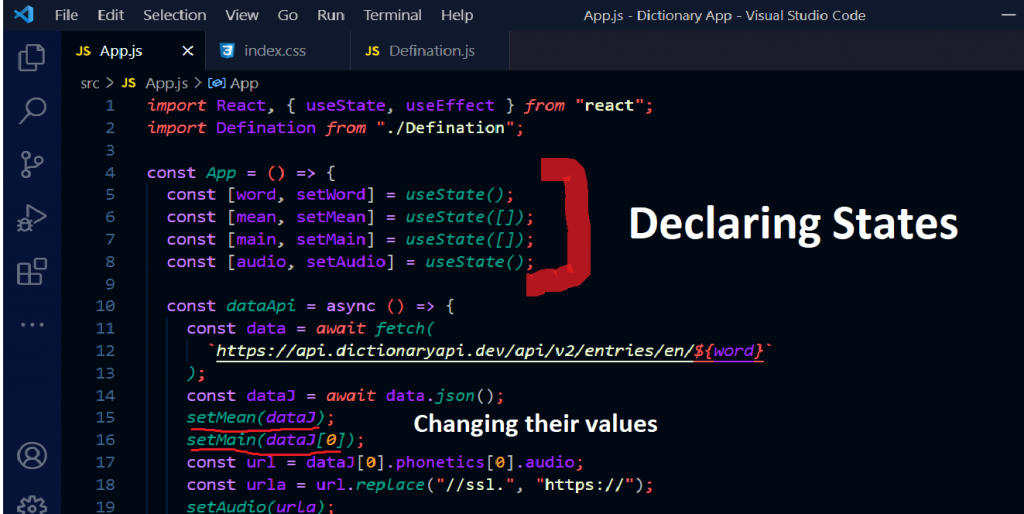
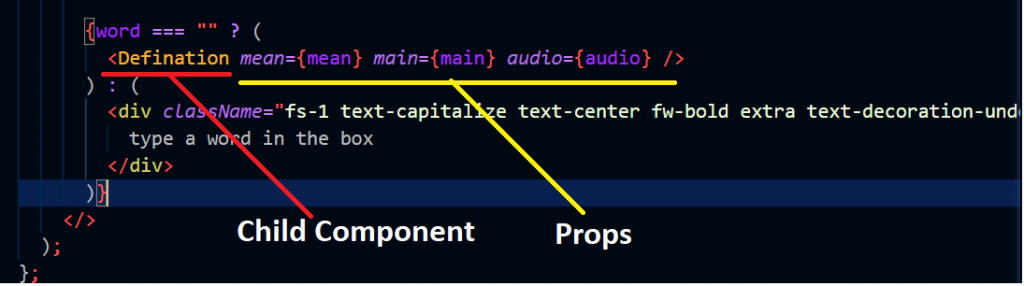
Now how are they called inside the child component?
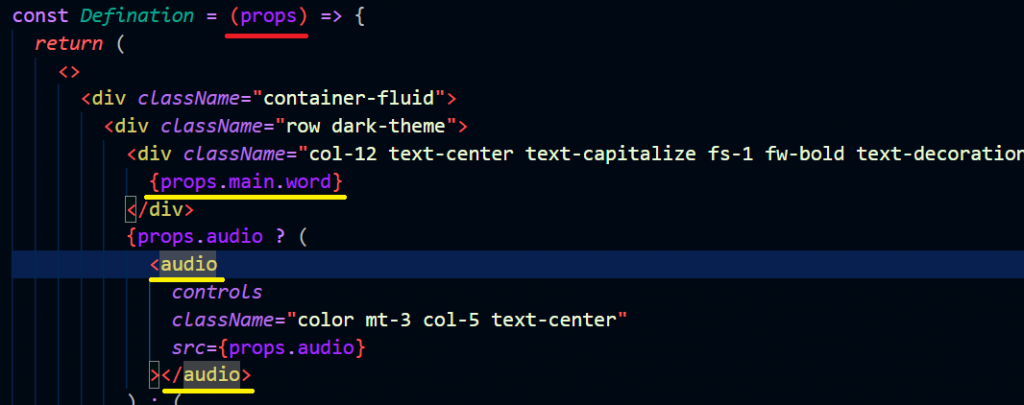
There is another way of calling props in the child component and that is by destructuring it.
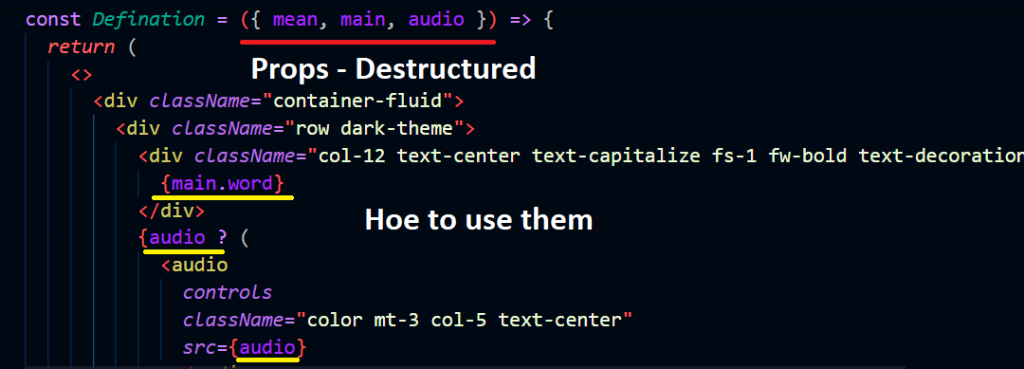
Let’s look at the differences between them in tabular form
Props | State |
Immutable – read-only | Mutable – can be changed |
Can be accessed among different components | Can only be accessed by the parent component – internally |
Now that we have understood the differences between props and states, let’s continue our topic and find out the differences between the functional and class components.
Difference between the functional and class components
Functional Components
Functional Components are JavaScript functions that are usually created with the help of arrow functions but can also be created without them.
Functional Components were called stateless Components as they did not have a way that can deal with state and were best suited for passing props and for developing the UI.
After the introduction of Hooks, they can deal with states and lifecycle methods, as they do not have their lifecycle methods.
Syntax
import React from 'react'
const App = () => {
return (
<>
</>
)
}
export default App
Class Components
They are usually called the stateful components in React as they can deal with states, which functional components were unable to do so before the introduction of Hooks.
They use the render methods and can even extend the class components.
They usually write “this” before any props or state and have their lifecycle methods.
Syntax
import React, { Component } from 'react'
export class App extends Component {
render() {
return (
<>
</>
)
}
}
export default App
As we have a basic idea of what functional and class components are. We will be seeing it in tabular form too –
Functional Components | Class Components |
Simple JS functions that return JSX | Simple JS class that extends its components and uses a render method |
No prefixes are required to use or to change the values of state and props | Prefix ‘this’ is added if you want to deal with state or props |
Hooks are used as their lifecycle methods as they don’t have their own | They have lifecycle methods available and are pretty much used like componentDiMount |
Let’s see them in action
Ex – Making a Counter that increases and decreases itself when the button is clicked
Using Functional Components
import React, {useState} from 'react';
const App = () => {
const[Input, setInput] = useState(0)
const Increase = () => {
setInput(Input + 1);
}
const Decrease = () => {
if(Input > 0) {
setInput(Input - 1);
}else{
setInput(0);
alert("Reached minimum value")
};
}
return(
<>
<div className="main">
<div className="text_area">{Input}</div>
<br /><br/>
<button className="one" onClick={Increase}>Increase</button>
<button className="two" onClick = {Decrease}>Decrease</button>
</div>
</>
)
}
export default App;
You can see the final result here
Using Class Components
import React from "react";
class ClassComponent extends React.Component{
constructor(){
super();
this.state={
count :0
};
this.increase=this.increase.bind(this);
}
increase(){
this.setState({count : this.state.count +1});
}
render(){
return (
<div style={{margin:'50px'}}>
<h1>Welcome to Geeks for Geeks </h1>
<h3>Counter App using Class Component : </h3>
<h2> {this.state.count}</h2>
<button onClick={this.increase}> Add</button>
</div>
)
}
}
export default ClassComponent;
The output will remain the same as this
Conclusion
There are pros and cons for everything, and they are no exception as we have discussed in this blog.
Though I would recommend the functional components as they are simple JavaScript codes that use ES6 classes like arrow functions, destructuring, etc, making it even easier to write and debug code.
While on the other hand, class components may be a bit confusing with their use of ‘this’ word with every state and props and also when we have to change the state.
And on top of that, the React team is also working on functional components to make it even better in the future but that doesn’t mean that they are boycotting class components.
Lastly, it’s up to you to decide whether to use class components or functional components or you can even write codes in React without using JSX.